Popup Menu Button Example
This time we are going to create a Flutter Popup Menu Button Example. It’s not simple but custom popup menu button in flutter . It is a popup menu with a text and a image. Almost all the medium scale applications have this feature. Popup menu button makes your app clean and creates the great user experience.
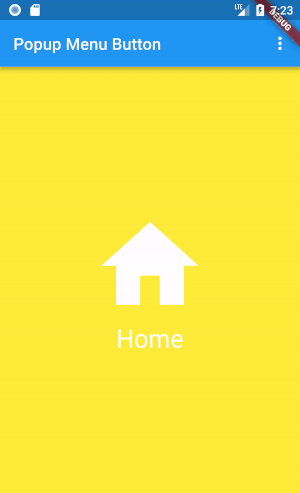
This is the main skull of Popup Menu Button . As you can see it is custom Popup Menu Button with class Custom Popup Menu. We will create that class and also onSelected we are calling to a function. We are going to code everything in a second.
import 'package:flutter/material.dart';
class Constants{
static const String Subscribe = 'Subscribe';
static const String Settings = 'Settings';
static const String SignOut = 'SignOut';
static const List <String> choices = <String>[
Subscribe,
Settings,
SignOut,
];
}
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Demo',
theme: new ThemeData(
primarySwatch: Colors.blue,
),
home: new MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text(widget.title),
actions: <Widget>[
PopupMenuButton<String>(
onSelected: choiceAction,
itemBuilder: (BuildContext context){
return Constants.choices.map((String choice){
return PopupMenuItem<String>(
value: choice,
child: Text(choice),
);
}).toList();
},
)
],
),
body: new Center(
child: new Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
new Text(
'Body',
),
],
),
),
);
}
void choiceAction(String choice){
if(choice == Constants.Settings){
print('Settings');
}else if(choice == Constants.Subscribe){
print('Subscribe');
}else if(choice == Constants.SignOut){
print('SignOut');
}
}
}
class Constants{
static const String Subscribe = 'Subscribe';
static const String Settings = 'Settings';
static const String SignOut = 'SignOut';
static const List <String> choices = <String>[
Subscribe,
Settings,
SignOut,
];
}
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Demo',
theme: new ThemeData(
primarySwatch: Colors.blue,
),
home: new MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text(widget.title),
actions: <Widget>[
PopupMenuButton<String>(
onSelected: choiceAction,
itemBuilder: (BuildContext context){
return Constants.choices.map((String choice){
return PopupMenuItem<String>(
value: choice,
child: Text(choice),
);
}).toList();
},
)
],
),
body: new Center(
child: new Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
new Text(
'Body',
),
],
),
),
);
}
void choiceAction(String choice){
if(choice == Constants.Settings){
print('Settings');
}else if(choice == Constants.Subscribe){
print('Subscribe');
}else if(choice == Constants.SignOut){
print('SignOut');
}
}
}
Comments